“Can’t talk now… I’m on a schedule.†“No, I have no time to meet this week.†“Really focused, can’t think about that right now.†If you have talked to me in the past two weeks, you have probably heard something like that. Also, you may have noticed that I have been a little slow on emails, not attending events that I normally go to (like the cypress coffee club networking meeting and the CyFair Chamber meetings or the Friday night chess meets, toastmasters, etc.) You may have noticed that the daily learn Hindi show on ISpeakHindi.com has been a little less than daily. And you might be tempted to ask “Are you OK?†or perhaps you think I am working on some super secret project. Well, it is time that I set the record straight.
These past two weeks I have been engaged in something that is best described as “continuous contact parentingâ€. In past summers we have sent the kinds off to various camps. But this summer I am at home and available to spend with them 24/7. I want to make the most of this time. Because I know that in less than a decade both of them will out of the house, and I will probably have time for them at that point, but they will probably be too busy with their lives for me.
But how to make the most of the summer? Should I just sit around and do whatever comes to mind. Should we just play board game after board game. Read books. Watch movies. What should we do?
I had some very specific ideas on what an ideal summer day might look like. It should have exercise. Time for eating. Piano practice. Math worksheets. Piano writing. But there is one area that I thought we could focus on and have a lot of fun: Electronics and robotics. After putting all that together, we came up with a daily schedule that we would try for two weeks:
The electronics time was spent learning about voltage, current, resistance, using the multi-meter, understanding the relationship between voltage, current, resistance, soldering, striping wires, reading circuit diagrams, making connections between components on a breadboard, using a Parallax Basic stamp.
Here are some pictures to give you some idea:
We also put together some kits:
In fact, we have a “wall of completed projects†where we put a postcard or something to indicate the projects that we have finished:
We have also programmed Parallax’s scribbler robot.
During our free time we did end up playing a board game, Settlers of Catan. My friend, Daniel Parker, in Austin introduced it to us a couple of weeks ago. Since then we have played dozens of games.
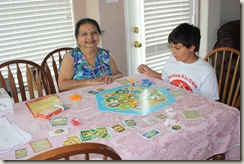
This is just a small glimpse of the things we have been up to.